();
-var certstreamClient = new CertstreamClient(ConnectionType.Full, logger: logger);
-await certstreamClient.StartAsync();
+ CertstreamClient client = new(ConnectionType.Full, logger: logger);
+ await client.StartAsync();
-certstreamClient.CertificateIssued += (sender, cert) =>
-{
- foreach (string domain in cert.AllDomains)
- {
- Console.WriteLine($"{cert.Issuer.O ?? cert.Issuer.CN} issued a SSL certificate for {domain}");
- }
-};
+ client.CertificateIssued += (sender, cert) =>
+ {
+ foreach (string domain in cert.AllDomains)
+ Console.WriteLine($"{cert.Issuer.O ?? cert.Issuer.CN} issued a SSL certificate for {domain}");
+ };
-Console.ReadKey();
+ Console.ReadKey(true);
-await certstreamClient.StopAsync();
+ await client.StopAsync();
-Console.ReadKey();
+ Console.ReadKey(true);
+ }
+ }
+}
\ No newline at end of file
diff --git a/README.md b/README.md
index 040ad21..8048418 100644
--- a/README.md
+++ b/README.md
@@ -1,11 +1,11 @@
# Certstream
-
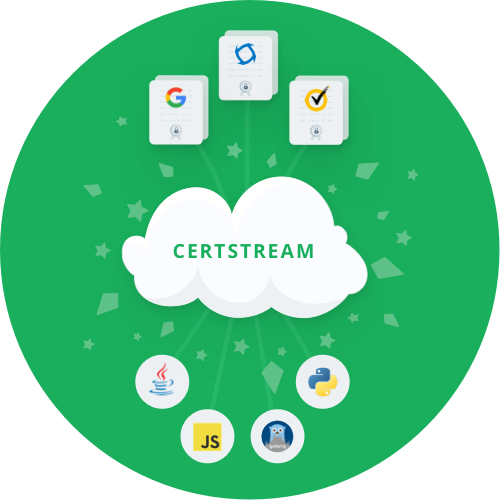
+
- A C# library for processing newly issued SSL certificates in real time using the Certstream API.
+ C# library for real-time SSL certificate processing using the Calidog Certstream API.
## Usage